Zero-Shot Text Classification in 8 Minutes: A Step-by-Step Guide
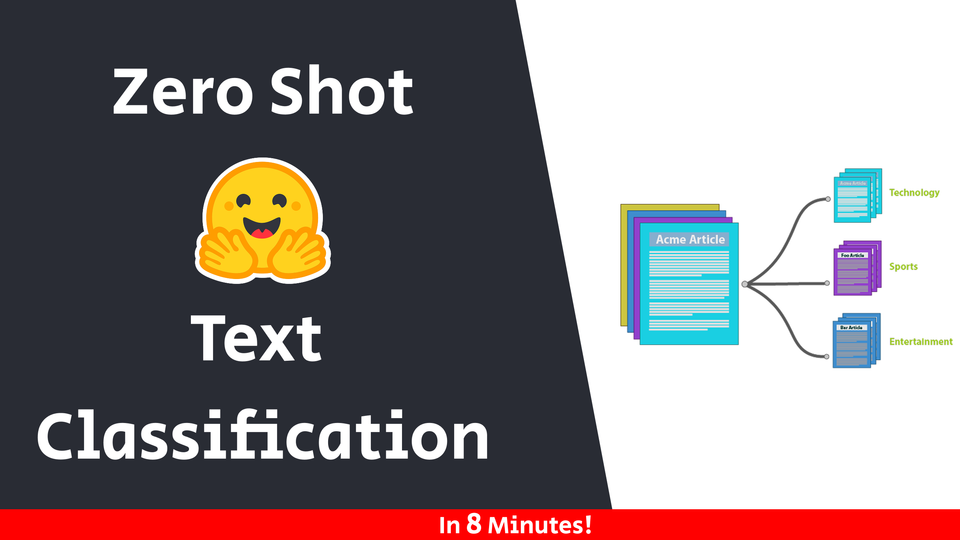
In today’s fast-paced world, efficiency is everything—even in machine learning. What if you could build a text classifier without spending hours on data labeling or training? In this blog post, we’ll explore zero-shot text classification, a powerful technique that allows you to classify text into any set of labels—without the need to train a model from scratch.
In this tutorial, I demonstrate how to use Hugging Face’s pre-trained model (facebook/bart-large-mnli
) to perform zero-shot classification. This approach is perfect for tasks such as sentiment analysis, content moderation, or topic categorization, and the best part is, you can see it all in action in just 8 minutes!
What Is Zero-Shot Learning?
Zero-shot learning is a method where a model can correctly predict or classify data that it has never seen before. Instead of training a model on a specific dataset, you leverage a model pre-trained on a large corpus of data—in our case, one fine-tuned for natural language inference (NLI). This means that you can simply provide the model with a list of candidate labels, and it will rank them according to how well they match the input text.
Why Use Zero-Shot Text Classification?
Traditional classification tasks require large, labeled datasets. However, obtaining and labeling data can be time-consuming and expensive. With zero-shot classification, you bypass the need for a dedicated training phase. This allows you to:
- Quickly prototype ideas: Test different labels or tasks without extensive preparation.
- Flexibly apply the model: Use it for various applications like sentiment analysis, topic detection, or content moderation.
- Save time and resources: No need to build and maintain large labeled datasets.
Setting Up Your Environment
Before diving into the code, ensure you have Python installed along with the Transformers library. You can install it via pip:
pip install transformers
Step-by-Step Code Walkthrough
Below is the complete code that you’ll see in the video, along with detailed explanations for each step.
1. Importing Libraries & Creating the Pipeline
We start by importing the necessary function and setting up our classifier.
from transformers import pipeline
# Create a zero-shot classifier pipeline using Facebook's BART model fine-tuned on MNLI.
classifier = pipeline("zero-shot-classification", model="facebook/bart-large-mnli")
Explanation:
- Importing
pipeline
: This high-level function from Hugging Face’s Transformers library simplifies many NLP tasks. - Setting up the pipeline: By specifying
"zero-shot-classification"
as the task and choosing thefacebook/bart-large-mnli
model, we’re leveraging a model trained for natural language inference (NLI). This model is adept at comparing a given text with candidate labels to determine the best match.
2. Defining the Input Text & Candidate Labels
Next, we define the text we want to classify and the labels against which the text will be evaluated.
# Example for Sentiment Analysis
sequence = "I absolutely love the new smartphone design – it’s sleek and super intuitive!"
candidate_labels = ["positive sentiment", "negative sentiment", "neutral"]
Explanation:
- Input Text: This is the text review we want to analyze.
- Candidate Labels: For this example, we’re classifying the sentiment of the text. The model will score each label based on how well it fits the input.
3. Running the Classification & Interpreting the Output
We then run the classifier with our sample text and candidate labels and print the result.
# Run the classifier and capture the result
result = classifier(sequence, candidate_labels)
# Print the output
print("Sentiment Analysis Result:")
print(result)
Explanation:
- Running the Classifier: The
classifier
function processes the input text along with the candidate labels. - Output Structure: The output is a dictionary that includes:
labels
: The candidate labels ranked from most to least relevant.scores
: Confidence scores for each label, indicating how likely the text belongs to that category.
- This quick output helps you instantly see which sentiment (or label) best matches the input text.
4. Variation: Topic Classification
Zero-shot classification isn’t limited to sentiment analysis. You can easily adapt the same code for topic categorization or any other task by changing the candidate labels.
# Example for Topic Classification
sequence_topic = "The recent advancements in artificial intelligence are transforming industries across the board."
candidate_topics = ["technology", "politics", "health", "sports"]
# Run the classifier for topic classification
result_topic = classifier(sequence_topic, candidate_topics)
print("\nTopic Classification Result:")
print(result_topic)
Explanation:
- Different Use Case: Here, we use a new sample text related to AI advancements.
- Candidate Topics: Instead of sentiment, we provide topic labels. The model evaluates which topic best describes the text.
- This demonstrates the flexibility of zero-shot classification: with a simple change in candidate labels, you can repurpose the same model for entirely different tasks.
Real-World Applications
Zero-shot text classification can be applied in many scenarios:
- Content Moderation: Automatically flagging or filtering content on social media or forums.
- Sentiment Analysis: Quickly gauging the sentiment of customer reviews or social media posts.
- Topic Categorization: Organizing news articles, blog posts, or research papers into predefined topics.
- Customer Feedback Analysis: Sorting feedback into categories like “feature request”, “bug report”, or “praise.”
By leveraging pre-trained models, you can experiment with and deploy these applications without the heavy lifting of data labeling and model training.
Conclusion
In this tutorial, we walked through how to implement zero-shot text classification using Hugging Face’s Transformers library. Here’s what we covered:
- Understanding Zero-Shot Learning: A method to classify text without the need for training on a dedicated dataset.
- Setting Up the Pipeline: How to use the
facebook/bart-large-mnli
model with a few simple lines of code. - Running and Interpreting the Model: A detailed code walkthrough for sentiment analysis and a quick variation for topic classification.
- Real-World Applications: How you can apply this technique in various scenarios to save time and resources.
Zero-shot classification is a powerful tool in your machine learning arsenal, offering a flexible and efficient way to tackle a range of text classification problems. I encourage you to try this out for your own projects and explore the endless possibilities it offers.
If you enjoyed this tutorial, please feel free to like, comment, and share your experiments. For more bite-sized machine learning tutorials and code walkthroughs, don’t forget to subscribe to my channel!