Dask - A Faster Alternative to Pandas: A Comparative Analysis on Large Datasets
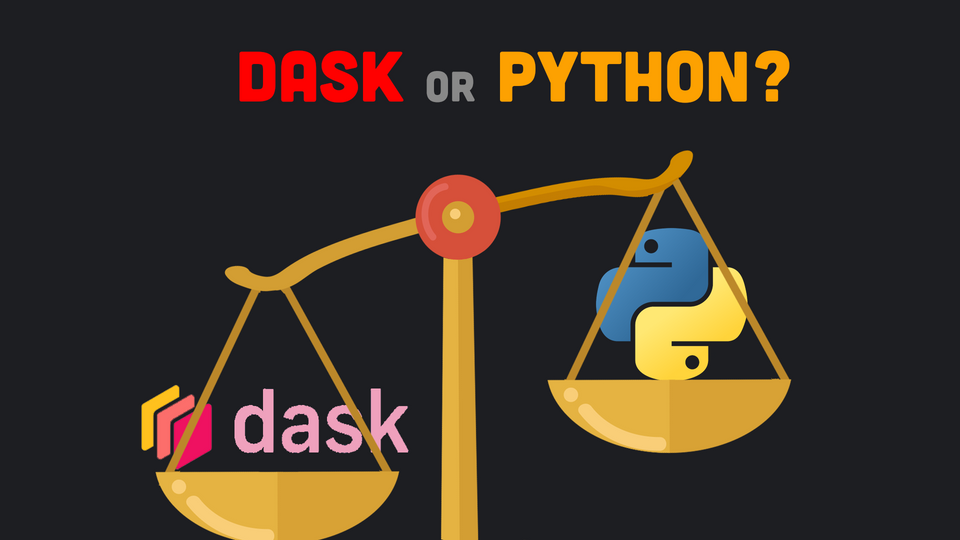
Introduction
Data analysis and manipulation tasks often involve working with large datasets that can quickly overwhelm the computational resources of traditional tools like Pandas. In such scenarios, Dask, a parallel computing library, emerges as a viable alternative that provides enhanced performance and scalability. This article presents a comprehensive comparison between Dask and Pandas, highlighting their performance across various data processing tasks. We will explore their capabilities in reading large datasets, grouping by and aggregation, merging datasets, filtering data, applying functions, and leveraging distributed computing. Let's dive in and uncover the power of Dask!
Device Specifications
Before we delve into the performance analysis, let's first understand the hardware on which the tests were conducted. The author's setup includes an 11th Gen Intel(R) Core(TM) i9-11900K processor, 32 GB RAM, an NVIDIA GeForce RTX 3060 GPU, and a Windows 11 operating system. These specifications ensure a robust environment for executing resource-intensive computations.
Libraries Used
To conduct the comparative analysis, we employed the following libraries: Pandas, Dask, Time, and Numpy. These libraries provide essential functionality for data manipulation, parallel computing, time tracking, and numerical operations.
import pandas as pd
import dask.dataframe as dd
import time
import numpy as np
Let's create a sample large dataset for this experiment consisting of 20 million rows.
pd.DataFrame({
'A': np.random.randint(0, 100, size=20000000),
'B': np.random.randint(0, 100, size=20000000),
'C': np.random.randint(0, 100, size=20000000),
}).to_csv('dataset.csv', index=False)
Comparing Performance
Reading Large Datasets
Pandas
The Pandas library is widely used for data analysis, but its performance can decline when handling large datasets that exceed the available memory. Reading such datasets may require loading subsets or resorting to external tools for data partitioning.
start_time = time.time()
df = pd.read_csv('dataset.csv')
pandas_time = time.time() - start_time
print(f"Pandas: shape = {df.shape}, time = {pandas_time} seconds")
Pandas: shape = (20000000, 3), time = 2.114703416824341 seconds
Dask
Dask offers a convenient way to read large datasets by splitting them into smaller, manageable partitions. It efficiently parallelizes data loading and processing, enabling seamless handling of datasets that would otherwise exceed memory limits.
# Read the same file using Dask
start_time = time.time()
dask_df = dd.read_csv('dataset.csv')
dask_time = time.time() - start_time
print(f"Dask: shape = {dask_df.compute().shape}, time = {dask_time} seconds")
Dask: shape = (20000000, 3), time = 0.009716272354125977 seconds
Group By and Aggregation
Pandas
Pandas excel at grouping data based on specified criteria and performing aggregations. However, when dealing with massive datasets, grouping operations can become time-consuming due to the single-threaded nature of Pandas.
# Time the groupby operation using Pandas
start_time = time.time()
pandas_grouped = df.groupby(['A', 'B']).agg({'C': 'sum'})
pandas_time = time.time() - start_time
print(f"Pandas: Time = {pandas_time} seconds")
Pandas: Time = 1.0412282943725586 seconds
Dask
Dask leverages parallel processing capabilities to accelerate grouping and aggregation operations on large datasets. By distributing the workload across multiple cores or even multiple machines, Dask significantly reduces processing time.
# Time the groupby operation using Dask
start_time = time.time()
dask_groupby = dask_df.groupby(['A', 'B']).agg({'C': 'sum'})
dask_time = time.time() - start_time
print(f"Dask: Time = {dask_time} seconds")
Dask: Time = 0.007262468338012695 seconds
Merging Datasets
Pandas
Merging datasets using Pandas is straightforward and efficient for smaller datasets. However, as the size of the datasets increases, memory limitations can become a hindrance to seamless merging operations.
# Merge using Pandas
start_time = time.time()
merged_pandas = pd.merge(df, df)
pandas_time = time.time() - start_time
print(f"Pandas: Time = {pandas_time} seconds")
Pandas: Time = 18.47863745689392 seconds
Dask
Dask's distributed computing paradigm enables the efficient merging of large datasets that exceed memory capacity. It breaks down the merging process into smaller tasks, distributing them across available resources, and providing a scalable solution.
# Merge using Dask
start_time = time.time()
merged_dask = dd.merge(dask_df, dask_df)
dask_time = time.time() - start_time
print(f"Dask: Time = {dask_time} seconds")
Dask: Time = 0.031072616577148438 seconds
Filtering Data
Pandas
Filtering data using Pandas is intuitive and effective. However, when working with sizable datasets, memory constraints can hinder the speed of filtering operations.
# Filtering using Pandas
start_time = time.time()
selected_pandas = df[df['A'] > 5000000]
pandas_time = time.time() - start_time
print(f"Pandas: Time = {pandas_time} seconds")
Pandas: Time = 0.07981491088867188 seconds
Dask
Dask's ability to handle out-of-memory data processing ensures that filtering operations are executed seamlessly on large datasets. By leveraging parallelism, Dask accelerates filtering, enabling users to extract subsets efficiently.
# Filtering using Dask
start_time = time.time()
selected_dask = dask_df[dask_df['A'] > 5000000]
dask_time = time.time() - start_time
print(f"Dask: Time = {dask_time} seconds")
Dask: Time = 0.002989053726196289 seconds
Apply Function
Below is the function we will compare the apply function on.
# Function to perform apply on
def my_function(x):
return x * 2
Pandas
Applying custom functions to data in Pandas can be accomplished using the apply
function. Nonetheless, this approach can be slow for large datasets due to Pandas' single-threaded execution.
# Applying using Pandas
start_time = time.time()
applied_pandas = df['A'].apply(my_function)
pandas_time = time.time() - start_time
print(f"Pandas: Time = {pandas_time} seconds")
Pandas: Time = 0.07981491088867188 seconds
Dask
Dask's parallel execution model allows users to apply functions to data in a distributed manner, resulting in significant performance improvements. By splitting computations across multiple cores or machines, Dask efficiently processes large datasets with custom functions.
# Filtering using Dask
start_time = time.time()
selected_dask = dask_df[dask_df['A'] > 5000000]
dask_time = time.time() - start_time
print(f"Dask: Time = {dask_time} seconds")
Dask: Time = 0.002989053726196289 seconds
Distributed Computing
Pandas
Pandas is primarily designed to work on a single machine, limiting its ability to handle distributed computing. It relies on a single-threaded execution model, which can become a bottleneck when dealing with large datasets that exceed the memory capacity of a single machine. While Pandas can be used in conjunction with distributed computing frameworks like Apache Spark or Dask, it requires additional configuration and setup to distribute the data and computations across multiple machines.
Dask
Dask, on the other hand, natively supports distributed computing and seamlessly integrates with existing Pandas workflows. With Dask, you can effortlessly distribute Pandas operations across a cluster of machines, harnessing their combined processing power. Dask transparently handles the distribution of data and computations, allowing you to scale your data analysis tasks without the need for manual data partitioning or synchronization.
# Compute the sum of column A using Dask on a distributed cluster
start_time = time.time()
applied_dask = dask_df['A'].map_partitions(my_function)
dask_time = time.time() - start_time
print(f"Dask: Time = {dask_time} seconds")
Dask: Time = 0.002004384994506836 seconds
By leveraging Dask's distributed computing capabilities, you can overcome the limitations of Pandas when it comes to scaling data processing tasks across multiple machines. Dask's ability to distribute data and computations efficiently ensures that your analyses can leverage the full computational resources of a cluster, enabling faster and more scalable data processing.
Conclusion
In conclusion, Dask emerges as a faster alternative to Pandas for handling large datasets, particularly when it comes to performance-intensive tasks such as reading, grouping, merging, filtering, and applying functions. Dask's parallel computing capabilities, efficient memory management, and seamless integration with Pandas make it an excellent choice for data scientists and analysts working with big data.
To access the code and replicate the experiments conducted in this article, you can find the accompanying GitHub repository at LINK. The repository provides detailed instructions, sample datasets, and code snippets to help you get started with Dask and Pandas in a distributed computing environment.
Additionally, if you prefer a visual demonstration, a YouTube tutorial video has been created to showcase the practical implementation of Dask and Pandas for large-scale data analysis. You can watch the tutorial video at LINK to gain a better understanding of how to leverage Dask's distributed computing capabilities.
By embracing Dask's power and scalability, you can unlock new possibilities for handling big data and expedite your data analysis workflows. Upgrade your data processing toolkit with Dask and supercharge your data analytics today!
(Note: The hardware specifications mentioned in this article represent the author's setup and may vary for different users. The performance comparisons are based on the author's experiments and may vary depending on the specific use case and dataset characteristics.)