Boost Your Image Models with Albumentations: A Quick 8-Minute Guide
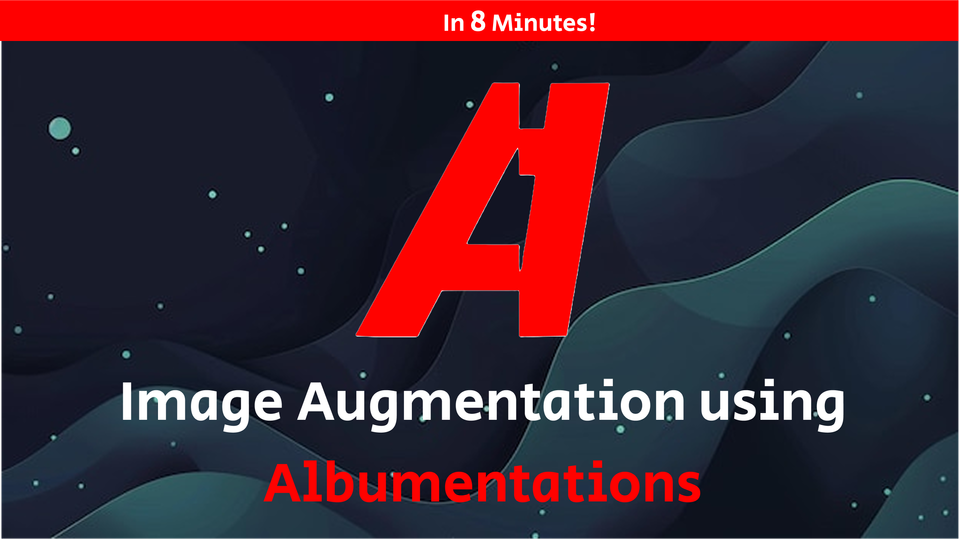
Welcome to this in-depth guide where we dive into the powerful world of image augmentation using the Albumentations library. In this blog post, we'll walk through everything you need to know—from installing Albumentations and setting up your environment, to building a transformation pipeline and visualizing the augmented images. Whether you're a beginner or an experienced practitioner looking to refresh your skills, this guide has you covered!
Introduction
Image augmentation is a critical component in training robust deep learning models for computer vision tasks. By applying a variety of transformations to your training data, you can help your model generalize better, reduce overfitting, and mimic different real-world scenarios. In this tutorial, we’ll be using the Albumentations library, which provides an easy-to-use interface for applying these augmentations in a fast and flexible manner.
In our accompanying video, “8-Minute Guide: Supercharge Your Image Models with Albumentations,” we show you how to get started quickly. In this blog post, we’ll elaborate on each step with detailed explanations and code snippets that you can run locally.
Why Image Augmentation?
Deep learning models typically require a lot of data to perform well. However, collecting and labeling massive datasets can be both time-consuming and expensive. Image augmentation addresses this challenge by:
- Increasing Data Diversity: Generate new training examples by altering existing images.
- Preventing Overfitting: Expose the model to varied data, reducing the likelihood of memorization.
- Simulating Real-World Variations: Mimic changes in lighting, orientation, and perspective that a model might encounter in production.
By integrating these techniques into your training pipeline, you can significantly boost your model’s performance.
Setting Up the Environment
Before diving into the code, ensure you have a Python environment ready. It’s recommended to use a virtual environment to manage dependencies. Then, install Albumentations using pip:
pip install albumentations
Along with Albumentations, we’ll need a few other libraries for this tutorial:
- OpenCV: For image loading and processing.
- Matplotlib: For visualization.
- Albumentations PyTorch Extension: To convert images into PyTorch tensors.
Here’s how to import the required libraries in your Python script or notebook:
import albumentations as A
from albumentations.pytorch import ToTensorV2
import cv2
import matplotlib.pyplot as plt
Explanation:
albumentations as A
makes it easy to reference the library.ToTensorV2
converts images to PyTorch tensors.cv2
is from OpenCV, used to load and manipulate images.matplotlib.pyplot
is used for plotting images side by side.
Building an Augmentation Pipeline
Now that our environment is set up, let's create a transformation pipeline. A pipeline is a sequence of augmentation operations that will be applied to each image.
Defining the Pipeline
Here’s an example of a simple pipeline using Albumentations:
transform = A.Compose([
A.HorizontalFlip(p=0.5),
A.RandomRotate90(p=0.5),
A.RandomBrightnessContrast(p=0.3),
A.Blur(blur_limit=3, p=0.3),
A.RandomCrop(width=256, height=256, p=0.3),
# Real World Training, Uncomment Below
# A.Normalize(mean=(0.485, 0.456, 0.406), std=(0.229, 0.224, 0.225)),
ToTensorV2()])
Explanation of Each Transformation:
- A.HorizontalFlip(p=0.5):
Randomly flips the image horizontally with a 50% chance. This helps your model learn that the orientation of objects may vary. - A.RandomRotate90(p=0.5):
Rotates the image by 90 degrees randomly with a 50% chance. This adds rotational invariance to your model. - A.RandomBrightnessContrast(p=0.2):
Adjusts the brightness and contrast of the image with a 20% probability, simulating different lighting conditions. - A.Blur(p=0.2):
Adds random blurring to the image with a 20% chance and a blur limit of 3. - A.RandomCrop(width=256, height=256, p=0.3):
Crops the Image randomly with a probability of 30% and a height and width of 256 x 256 pixels. - A.Normalize(...):
Normalizes the image using the mean and standard deviation values (commonly used for pretrained models like those on ImageNet). This standardizes your input data. - ToTensorV2():
Converts the image into a PyTorch tensor, which is necessary for model training in PyTorch.
Integration Tip:
This pipeline can be seamlessly integrated into your data loader, ensuring that every image is augmented on the fly during training.
Complete List of Albumentations Transforms - https://explore.albumentations.ai/
Visualizing the Augmentations
It’s essential to visualize the effects of your augmentations to verify that they’re being applied correctly. Below is a function that loads an image, applies the transformation multiple times, and displays both the original and augmented images side by side.
Loading an Image
First, load an image using OpenCV. Remember, OpenCV loads images in BGR format, so we need to convert it to RGB for proper visualization:
image = cv2.imread('sample.jpg')
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
Visualization Function
Now, let’s create a function to display a grid of images:
def visualize_transform(image, transform, num_examples=5):
fig, axes = plt.subplots(2, num_examples, figsize=(15, 6))
for i in range(num_examples):
augmented = transform(image=image)['image']
# Plot original image in the top row
axes[0, i].imshow(image)
axes[0, i].set_title("Original")
axes[0, i].axis('off')
# Plot augmented image in the bottom row
# If the augmented image is a tensor, convert it for display
if hasattr(augmented, 'permute'):
aug_img = augmented.permute(1, 2, 0).numpy()
else:
aug_img = augmented
axes[1, i].imshow(aug_img)
axes[1, i].set_title("Augmented")
axes[1, i].axis('off')
plt.tight_layout()
plt.show()
Explanation:
- Grid Setup:
We create a 2-row grid usingplt.subplots()
, where the top row displays the original image and the bottom row shows the augmented versions. - Image Conversion:
The function checks if the output is a tensor. If it is, it rearranges the dimensions using.permute()
and converts it to a numpy array for proper display. - Visualization:
The function loops through a specified number of examples (default is 5), applying the transformation each time to showcase the variety introduced by the augmentations.
Running the Visualization
Finally, run the function to see the results:
visualize_transform(image, transform, num_examples=5)
This code will display a grid with the original image on the top row and multiple augmented versions on the bottom row. Visualizing the effects is crucial to ensure that your augmentation pipeline is working as intended before you integrate it into your training loop.
Conclusion
In this guide, we covered the essentials of using Albumentations to enhance your image data for deep learning tasks. Here’s a quick recap:
- Installation and Setup:
We installed Albumentations and imported necessary libraries. - Building the Pipeline:
We created a robust augmentation pipeline using various transformations like horizontal flips, rotations, brightness/contrast adjustments, and normalization. - Visualization:
We implemented a visualization function to compare the original image with its augmented counterparts, ensuring our transformations are applied correctly.
By integrating these augmentation techniques into your training pipeline, you can improve your model’s robustness and performance, even when working with limited data.
What’s Next?
Try experimenting with additional transformations and fine-tune the probabilities to see how they affect your model’s performance. Don’t forget to check out the accompanying video for a quick, visual demonstration of these steps in action!
Happy Augmenting!